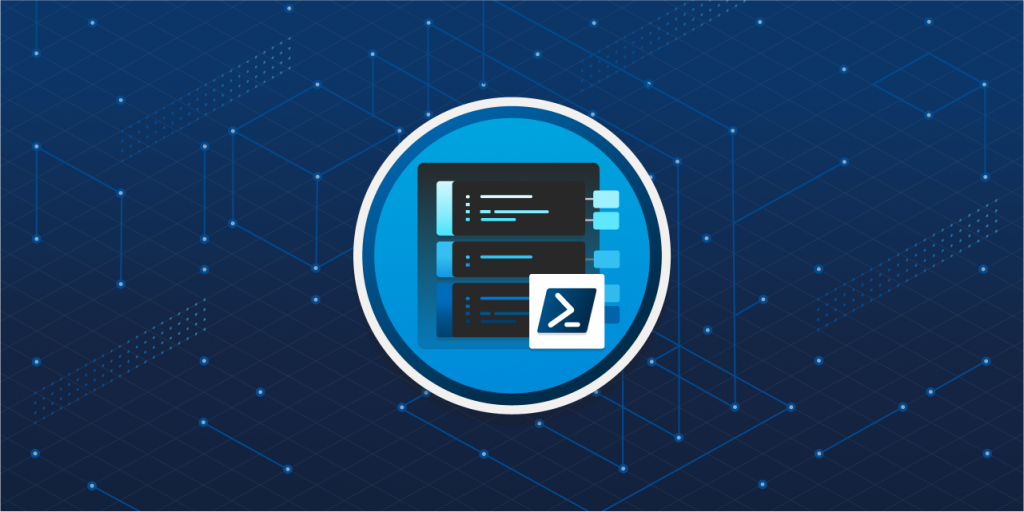
DHCP server implementation, configuration, and management are essential to automate IP address management within a network. PowerShell can be used to install, configure, and manage DHCP servers efficiently.
In this article, we will take a comprehensive look at how to use PowerShell to deploy and manage DHCP servers.
Install DHCP Server Role
Install-WindowsFeature -Name DHCP -IncludeManagementTools
Authorize DHCP Server in Active Directory:
Adding the Server to trusted DHCP servers and adding the DHCP security group
Add-DhcpServerInDC
Add-DHCPServerSecurityGroup
Update the registry to mark DHCP configuration as complete.
Set-ItemProperty -Path 'HKLM:\SOFTWARE\Microsoft\ServerManager\Roles\12' -Name 'ConfigurationState' -Value 2
Restart the DHCP server service to apply changes.
Restart-Service -Name DHCPServer -Force
Check DHCP Service Status
To display detailed information about the DHCP service status:
Get-Service -Name DHCPServer | Format-List -Property *
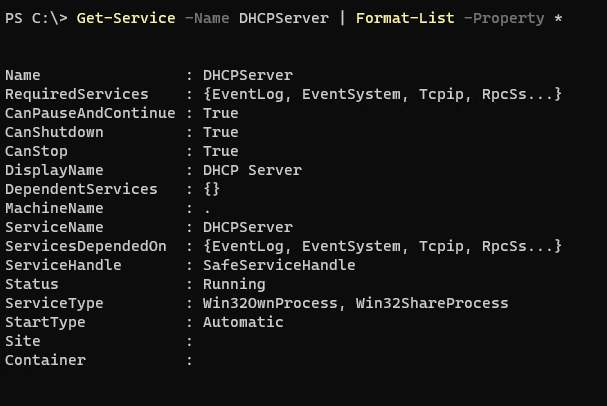
Configuring DHCP Scopes and Options:
Setting up a DHCP server in Windows Server is a straightforward process, but to make it functional, you need to configure DHCP scopes and options.
First, import the DHCP Server module in PowerShell. This module allows you to manage DHCP settings.
Import-Module DHCPServer -WarningAction SilentlyContinue
Create IPv4 Scopes:
Now we can create a new DHCP scope for a specific subnet. In this example, we’re creating a scope for the 192.168.1.0/24
 subnet.
$SCOPEHT = @{
Name = 'OfficeNetwork'
StartRange = '192.168.16.100'
EndRange = '192.168.16.200'
SubnetMask = '255.255.255.0'
ComputerName = 'DHCP01.Office.Local'
}
Add-DhcpServerV4Scope @SCOPEHT
View the DHCP Scopes:
To verify the scope creation, we can retrieve the list of DHCP scopes on the server.
Get-DhcpServerv4Scope -ComputerName DHCP01.Office.Local
Set Server-Wide DHCP Options:
We can also set options that apply to all scopes on the DHCP server. For example, configure the DNS domain and DNS server.
$OPTION1HT = @{
ComputerName = 'DHCP01.Office.Local'
DnsDomain = 'Office.Local'
DnsServer = '192.168.16.10'
}
Set-DhcpServerV4OptionValue @OPTION1HT
View DHCP Server Options:
To see the server-wide options, use the following command:
Get-DhcpServerv4OptionValue | Format-Table -AutoSize
View Scope-Specific Options:
To view options specific to a scope, use:
Get-DhcpServerv4OptionValue -ScopeId '192.168.16.0' | Format-Table -AutoSize
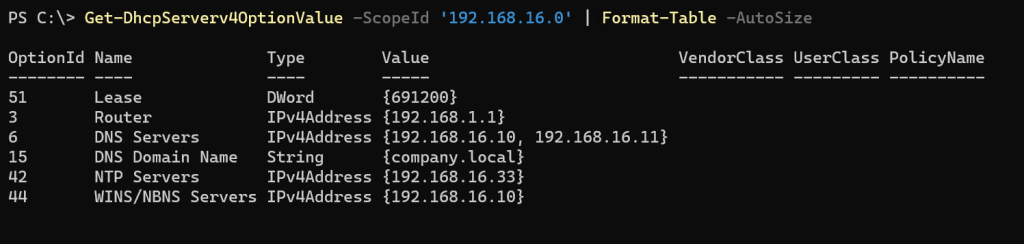
View DHCP Option Definitions:
Windows Server includes predefined DHCP options. To view them, run:
Get-DhcpServerv4OptionDefinition | Format-Table -AutoSize
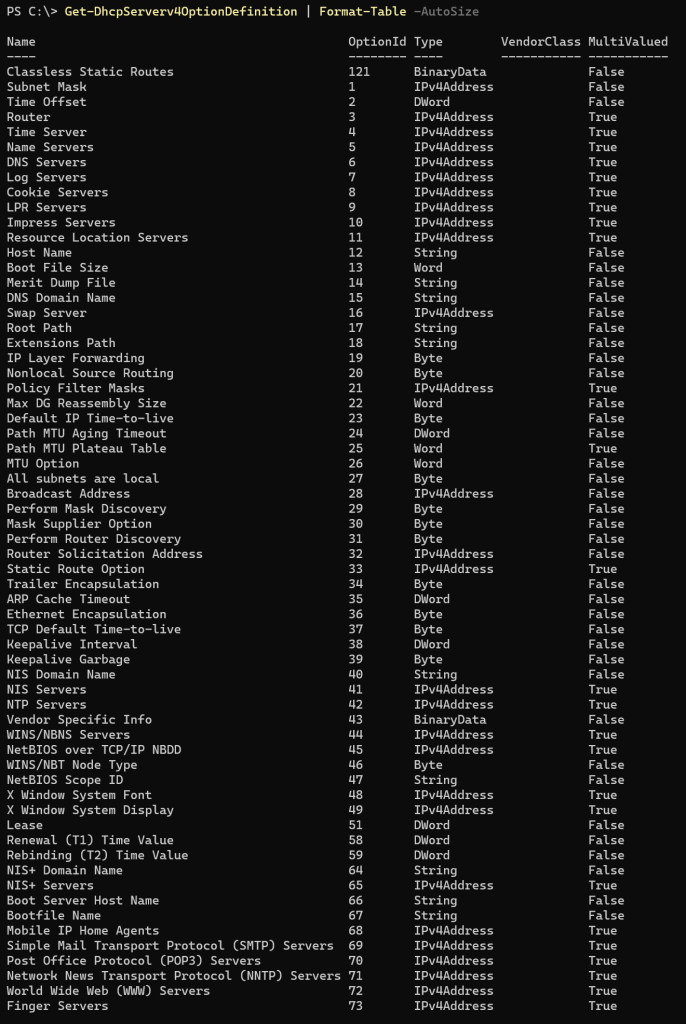
Check DHCP Scope Statistics:
View statistics for the DHCP scope to see how many addresses are in use and available.
Get-DhcpServerv4ScopeStatistics -ComputerName DHCP01
To list all leases (both active and reserved) in a specific scope, EXAMPLE:
Get-DhcpServerv4Lease -ScopeId '192.168.1.0'
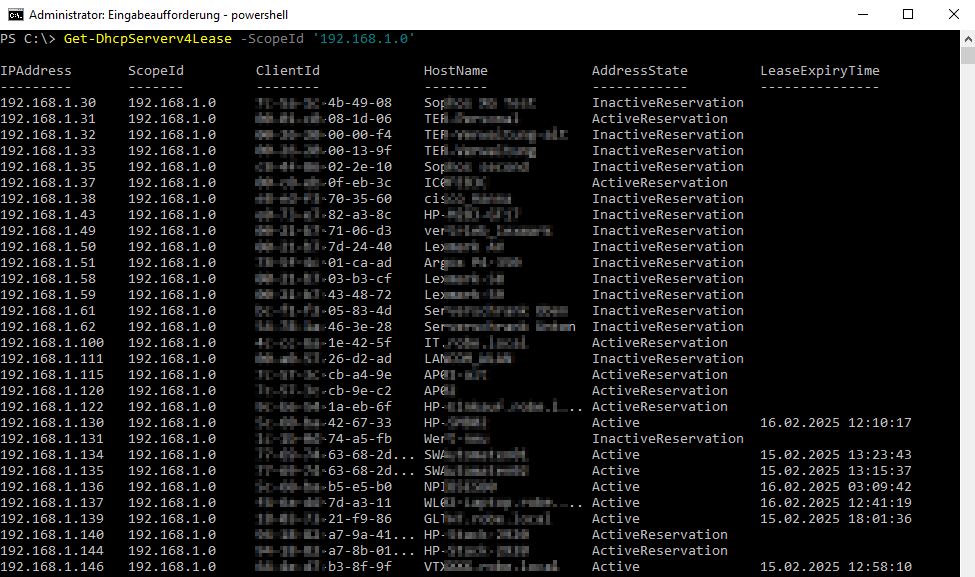
To list all reservations for a specific scope, EXAMPLE:
Get-DhcpServerv4Reservation -ScopeId '192.168.1.0'
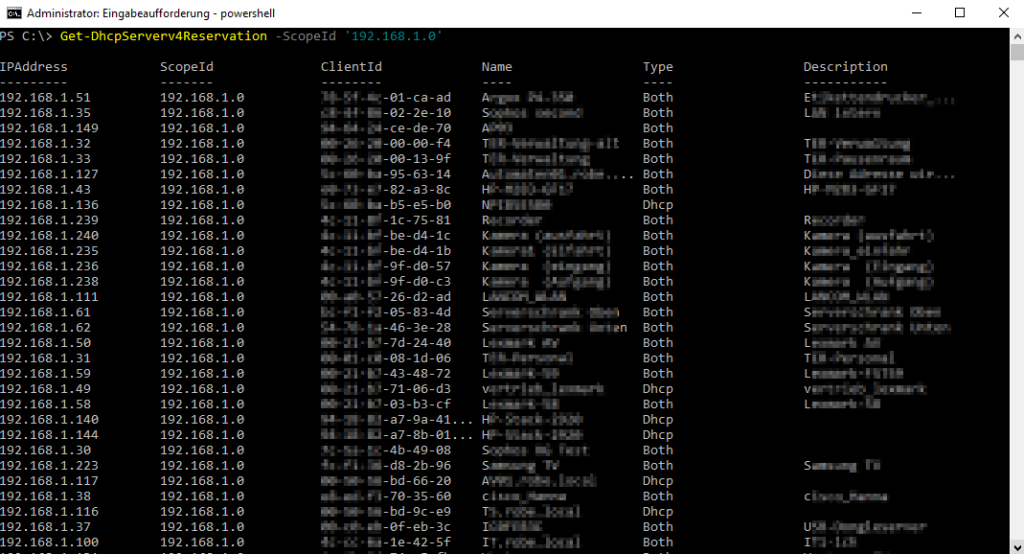
To find the next available IP address in the DHCP scope:
Get-DhcpServerv4FreeIPAddress -ComputerName DHCP01 -ScopeId '192.168.1.0'
Create a DHCP Reservation:
Add-DhcpServerv4Reservation -ScopeId '192.168.1.0' -IPAddress '192.168.1.199' -ClientId 'XX-XX-XX-XX-XX-XX' -ComputerName 'DHCP01'
For windows machines, you can try to find the MAC address of the network adapter like:
$SB = {Get-NetAdapter -Name 'Ethernet'}
$Nic = Invoke-command -ComputerName SRV3 -ScriptBlock $SB
$MAC = $Nic.MacAddress
If you do not know the adapter name, you can create a function that retrieves all NICs from the remote computers
function Get-RemoteNics {
param (
[string]$ComputerName
)
try {
$scriptBlock = { Get-NetAdapter }
$counter = 1
$nics = Invoke-Command -ComputerName $ComputerName -ScriptBlock $scriptBlock -ErrorAction Stop | ForEach-Object {
[PSCustomObject]@{
Index = $counter
Name = $_.Name
MacAddress = $_.MacAddress
}
$counter++
}
return $nics
}
catch {
Write-Error "Error retrieving NICs from $ComputerName : $($_.Exception.Message)"
return $null
}
}
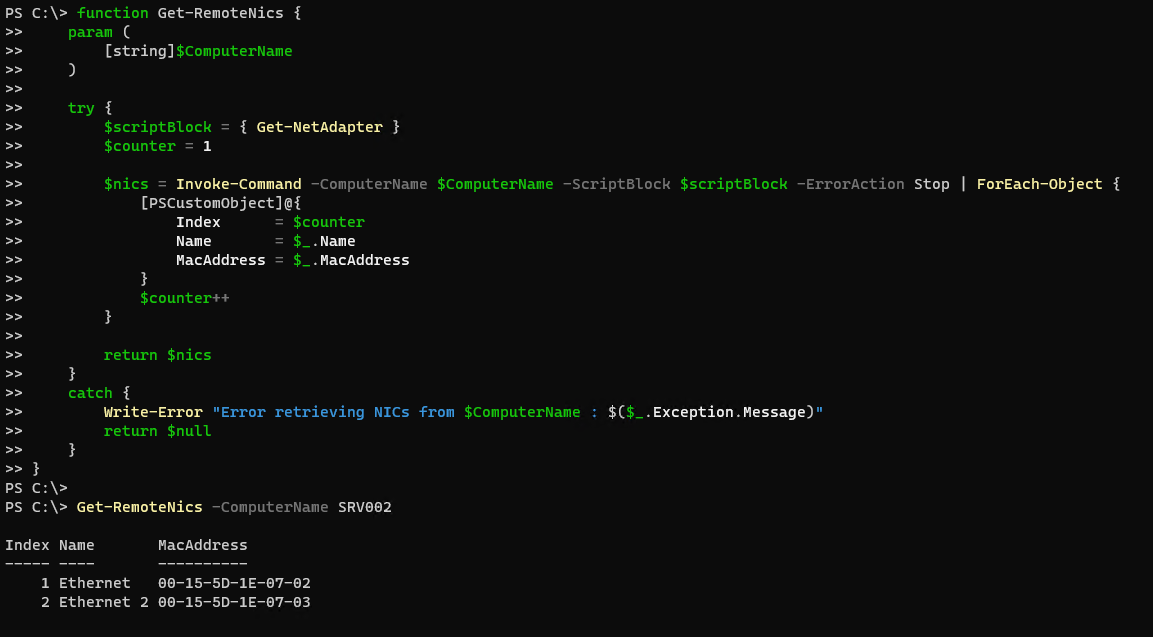
After obtaining the MAC address, you can create the DHCP reservation:
$NewResHT = @{
ScopeId = '192.168.1.0'
IPAddress = '192.168.1.199'
ClientId = $MAC
ComputerName = 'DHCP01'
}
Add-DhcpServerv4Reservation @NewResHT
Delete a DHCP Reservation:
Remove-DhcpServerv4Reservation -ScopeId '192.168.1.0' -ClientId 'XX-XX-XX-XX-XX-XX' -ComputerName 'DHCP01'
Putting It All Together
Here’s an example script to manage and configure a DHCP server on a Windows server machine. This script offers a simple menu interface for performing various DHCP-related tasks. You can easily customize it to suit your specific needs and requirements.
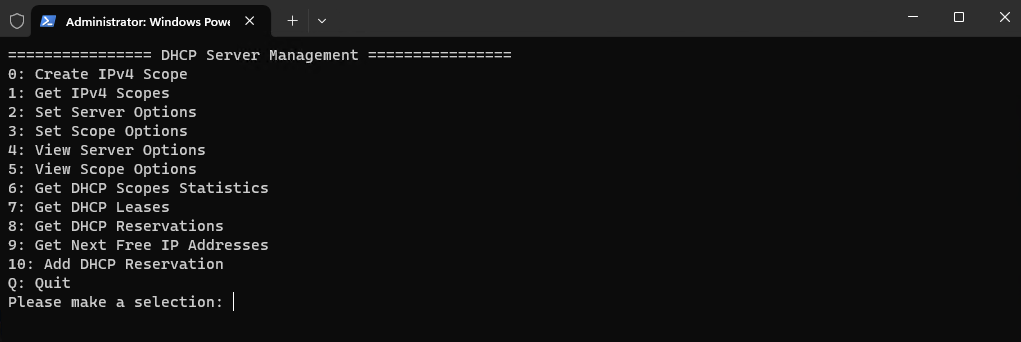
$logFile = "C:\DHCP_Mgmt_log.log"
function Log-Message {
param (
[string]$Message,
[string]$Level = "INFO"
)
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
$logEntry = "[$timestamp] [$Level] $Message"
Add-Content -Path $logFile -Value $logEntry
Write-Host $logEntry
}
function Test-DHCPServerModule {
if (!(Get-Module -ListAvailable -Name DHCPServer)) {
return $false
}
return $true
}
function Install-DHCP {
Log-Message "Installing DHCP feature..."
try {
Import-Module -Name ServerManager -WarningAction SilentlyContinue
Install-WindowsFeature -Name DHCP -IncludeManagementTools -ErrorAction Stop
Add-DhcpServerInDC -ErrorAction Stop
Add-DHCPServerSecurityGroup -ErrorAction Stop
Set-ItemProperty -Path 'HKLM:\SOFTWARE\Microsoft\ServerManager\Roles\12' -Name 'ConfigurationState' -Value 2 -ErrorAction Stop
Restart-Service -Name DHCPServer -Force -ErrorAction Stop
Get-Service -Name DHCPServer | Format-List -Property *
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
Log-Message "DHCP feature installed successfully."
}
catch {
Log-Message "Error installing DHCP feature: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-FQDN {
$computerSystem = Get-WmiObject -Class Win32_ComputerSystem
return "$($computerSystem.DNSHostName).$($computerSystem.Domain)"
}
function Create-IPv4Scope {
param (
[string]$ScopeName,
[string]$StartRange,
[string]$EndRange,
[string]$SubnetMask
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
$SCOPEHT = @{
Name = $ScopeName
StartRange = $StartRange
EndRange = $EndRange
SubnetMask = $SubnetMask
ComputerName = $ComputerName
}
Add-DhcpServerV4Scope @SCOPEHT -ErrorAction Stop
Log-Message "Scope $ScopeName created successfully."
}
catch {
Log-Message "Error creating scope $ScopeName : $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-IPv4Scopes {
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4Scope -ComputerName $ComputerName | Format-Table -AutoSize
Log-Message "Retrieved IPv4 scopes successfully."
}
catch {
Log-Message "Error retrieving IPv4 scopes: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Set-ServerOptions {
param (
[string]$DnsDomain,
[string]$DnsServer
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
if (-not ($DnsServer -match '\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b')) {
Log-Message "Invalid DNS server: $DnsServer. Please enter a valid IP address." -Level "ERROR"
return
}
$OPTIONHT = @{
ComputerName = $ComputerName
DnsDomain = $DnsDomain
DnsServer = $DnsServer
}
Set-DhcpServerV4OptionValue @OPTIONHT -ErrorAction Stop
Log-Message "Server-wide options set successfully."
}
catch {
Log-Message "Error setting server options: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Set-ScopeOptions {
param (
[string]$ScopeID,
[string]$Router,
[string[]]$DnsServers,
[string]$DnsDomain,
[string[]]$NtpServers,
[string[]]$WinsServers
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
if ($Router) {
Set-DhcpServerV4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID -OptionId 3 -Value $Router -ErrorAction Stop
}
if ($DnsServers) {
$DnsServersArray = $DnsServers -split ','
foreach ($DnsServer in $DnsServersArray) {
if ($DnsServer -match '\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b') {
Set-DhcpServerV4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID -OptionId 6 -Value $DnsServer -ErrorAction Stop
} else {
Log-Message "Invalid DNS server: $DnsServer" -Level "ERROR"
}
}
}
if ($DnsDomain) {
Set-DhcpServerV4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID -OptionId 15 -Value $DnsDomain -ErrorAction Stop
}
if ($NtpServers) {
$NtpServersArray = $NtpServers -split ','
foreach ($NtpServer in $NtpServersArray) {
if ($NtpServer -match '\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b') {
Set-DhcpServerV4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID -OptionId 42 -Value $NtpServer -ErrorAction Stop
} else {
Log-Message "Invalid NTP server: $NtpServer" -Level "ERROR"
}
}
}
if ($WinsServers) {
$WinsServersArray = $WinsServers -split ','
foreach ($WinsServer in $WinsServersArray) {
if ($WinsServer -match '\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b') {
Set-DhcpServerV4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID -OptionId 44 -Value $WinsServer -ErrorAction Stop
} else {
Log-Message "Invalid WINS server: $WinsServer" -Level "ERROR"
}
}
}
Log-Message "Scope-specific options set successfully for scope $ScopeID."
}
catch {
Log-Message "Error setting scope options for scope $ScopeID : $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function View-ServerOptions {
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4OptionValue -ComputerName $ComputerName | Format-Table -AutoSize
Log-Message "Retrieved server options successfully."
}
catch {
Log-Message "Error retrieving server options: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function View-ScopeOptions {
param (
[string]$ScopeID
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4OptionValue -ComputerName $ComputerName -ScopeId $ScopeID | Format-Table -AutoSize
Log-Message "Retrieved scope options successfully for scope $ScopeID."
}
catch {
Log-Message "Error retrieving scope options for scope $ScopeID : $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-DhcpScopeStatistics {
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4ScopeStatistics -ComputerName $ComputerName | Format-Table -AutoSize
Log-Message "Retrieved DHCP scope statistics successfully."
}
catch {
Log-Message "Error retrieving DHCP scope statistics: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-DhcpLeases {
param (
[string]$ScopeID
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4Lease -ComputerName $ComputerName -ScopeId $ScopeID | Format-Table -AutoSize
Log-Message "Retrieved DHCP leases successfully for scope $ScopeID."
}
catch {
Log-Message "Error retrieving DHCP leases: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-DhcpReservations {
param (
[string]$ScopeID
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4Reservation -ComputerName $ComputerName -ScopeId $ScopeID | Format-Table -AutoSize
Log-Message "Retrieved DHCP reservations successfully for scope $ScopeID."
}
catch {
Log-Message "Error retrieving DHCP reservations: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-DhcpFreeIPAddresses {
param (
[string]$ScopeID
)
try {
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
$ComputerName = Get-FQDN
Get-DhcpServerv4FreeIPAddress -ComputerName $ComputerName -ScopeId $ScopeID | Format-Table -AutoSize
Log-Message "Retrieved free IP addresses successfully for scope $ScopeID."
}
catch {
Log-Message "Error retrieving free IP addresses: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Get-RemoteNics {
param (
[string]$ComputerName
)
try {
$SB = { Get-NetAdapter }
$Nics = Invoke-Command -ComputerName $ComputerName -ScriptBlock $SB -ErrorAction Stop
return $Nics
}
catch {
Log-Message "Error retrieving NICs from $ComputerName : $($_.Exception.Message)" -Level "ERROR"
return $null
}
}
function Add-DhcpReservation {
param (
[string]$ScopeID,
[string]$IPAddress,
[string]$TargetComputerName
)
try {
$DHCPServerName = Get-FQDN
$Nics = Get-RemoteNics -ComputerName $TargetComputerName
if ($Nics -eq $null -or $Nics.Count -eq 0) {
Log-Message "No NICs found on $TargetComputerName. Reservation not created." -Level "ERROR"
return
}
Write-Host "Select a NIC to apply the reservation:"
$i = 1
$Nics | ForEach-Object {
Write-Host "$i : $($_.Name) - MAC: $($_.MacAddress)"
$i++
}
$Selection = Read-Host "Enter the number of the NIC to reserve"
$SelectedNic = $Nics[$Selection - 1]
$ClientId = $SelectedNic.MacAddress
$NewResHT = @{
ScopeId = $ScopeID
IPAddress = $IPAddress
ClientId = $ClientId
ComputerName = $DHCPServerName
}
Import-Module DHCPServer -WarningAction SilentlyContinue -ErrorAction Stop
Add-DhcpServerv4Reservation @NewResHT -ErrorAction Stop
Log-Message "DHCP reservation created successfully for IP $IPAddress on NIC $($SelectedNic.Name) with MAC $ClientId."
}
catch {
Log-Message "Error creating DHCP reservation: $($_.Exception.Message)" -Level "ERROR"
throw
}
}
function Show-Menu {
param (
[string]$Title = 'DHCP Server Management'
)
Clear-Host
Write-Host "================ $Title ================"
Write-Host "0: Create IPv4 Scope"
Write-Host "1: Get IPv4 Scopes"
Write-Host "2: Set Server Options"
Write-Host "3: Set Scope Options"
Write-Host "4: View Server Options"
Write-Host "5: View Scope Options"
Write-Host "6: Get DHCP Scopes Statistics"
Write-Host "7: Get DHCP Leases"
Write-Host "8: Get DHCP Reservations"
Write-Host "9: Get Next Free IP Addresses"
Write-Host "10: Add DHCP Reservation"
Write-Host "Q: Quit"
}
if (-not (Test-DHCPServerModule)) {
Log-Message "DHCP module not found." -Level "ERROR"
$response = Read-Host "Would you like to install the DHCP feature? (Y/N)"
if ($response -eq 'Y') {
try {
Install-DHCP
}
catch {
Log-Message "Script exiting due to error." -Level "ERROR"
exit
}
} else {
Log-Message "Script exiting. DHCP feature not installed." -Level "INFO"
exit
}
}
do {
Show-Menu
$selection = Read-Host "Please make a selection"
switch ($selection) {
'0' {
$ScopeName = Read-Host "Enter Scope Name"
$StartRange = Read-Host "Enter Start Range"
$EndRange = Read-Host "Enter End Range"
$SubnetMask = Read-Host "Enter Subnet Mask"
try {
Create-IPv4Scope -ScopeName $ScopeName -StartRange $StartRange -EndRange $EndRange -SubnetMask $SubnetMask
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'1' {
try {
Get-IPv4Scopes
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'2' {
$DnsDomain = Read-Host "Enter DNS Domain"
$DnsServer = Read-Host "Enter DNS Server"
try {
Set-ServerOptions -DnsDomain $DnsDomain -DnsServer $DnsServer
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'3' {
$ScopeID = Read-Host "Enter Scope ID"
$Router = Read-Host "Enter Router (optional)"
$DnsServers = (Read-Host "Enter DNS Servers (comma separated, optional)") -split ','
$DnsDomain = Read-Host "Enter DNS Domain (optional)"
$NtpServers = (Read-Host "Enter NTP Servers (comma separated, optional)") -split ','
$WinsServers = (Read-Host "Enter WINS Servers (comma separated, optional)") -split ','
try {
Set-ScopeOptions -ScopeID $ScopeID -Router $Router -DnsServers $DnsServers -DnsDomain $DnsDomain -NtpServers $NtpServers -WinsServers $WinsServers
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'4' {
try {
View-ServerOptions
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'5' {
$ScopeID = Read-Host "Enter Scope ID"
try {
View-ScopeOptions -ScopeID $ScopeID
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'6' {
try {
Get-DhcpScopeStatistics
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'7' {
$ScopeID = Read-Host "Enter Scope ID"
try {
Get-DhcpLeases -ScopeID $ScopeID
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'8' {
$ScopeID = Read-Host "Enter Scope ID"
try {
Get-DhcpReservations -ScopeID $ScopeID
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'9' {
$ScopeID = Read-Host "Enter Scope ID"
try {
Get-DhcpFreeIPAddresses -ScopeID $ScopeID
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'10' {
$ScopeID = Read-Host "Enter Scope ID"
$IPAddress = Read-Host "Enter IP Address"
$TargetComputerName = Read-Host "Enter Target Computer Name"
try {
Add-DhcpReservation -ScopeID $ScopeID -IPAddress $IPAddress -TargetComputerName $TargetComputerName
Read-Host "Press Enter to continue..."
}
catch {
Log-Message "Script paused due to error." -Level "ERROR"
Read-Host "Press Enter to continue..."
}
}
'Q' { break }
default { Write-Host "Invalid selection. Please try again." }
}
} while ($selection -ne 'Q')
You can download or run this script directly from here:
iex (irm https://yh.do/dhcpmgmt.ps1)
Leave a Comment